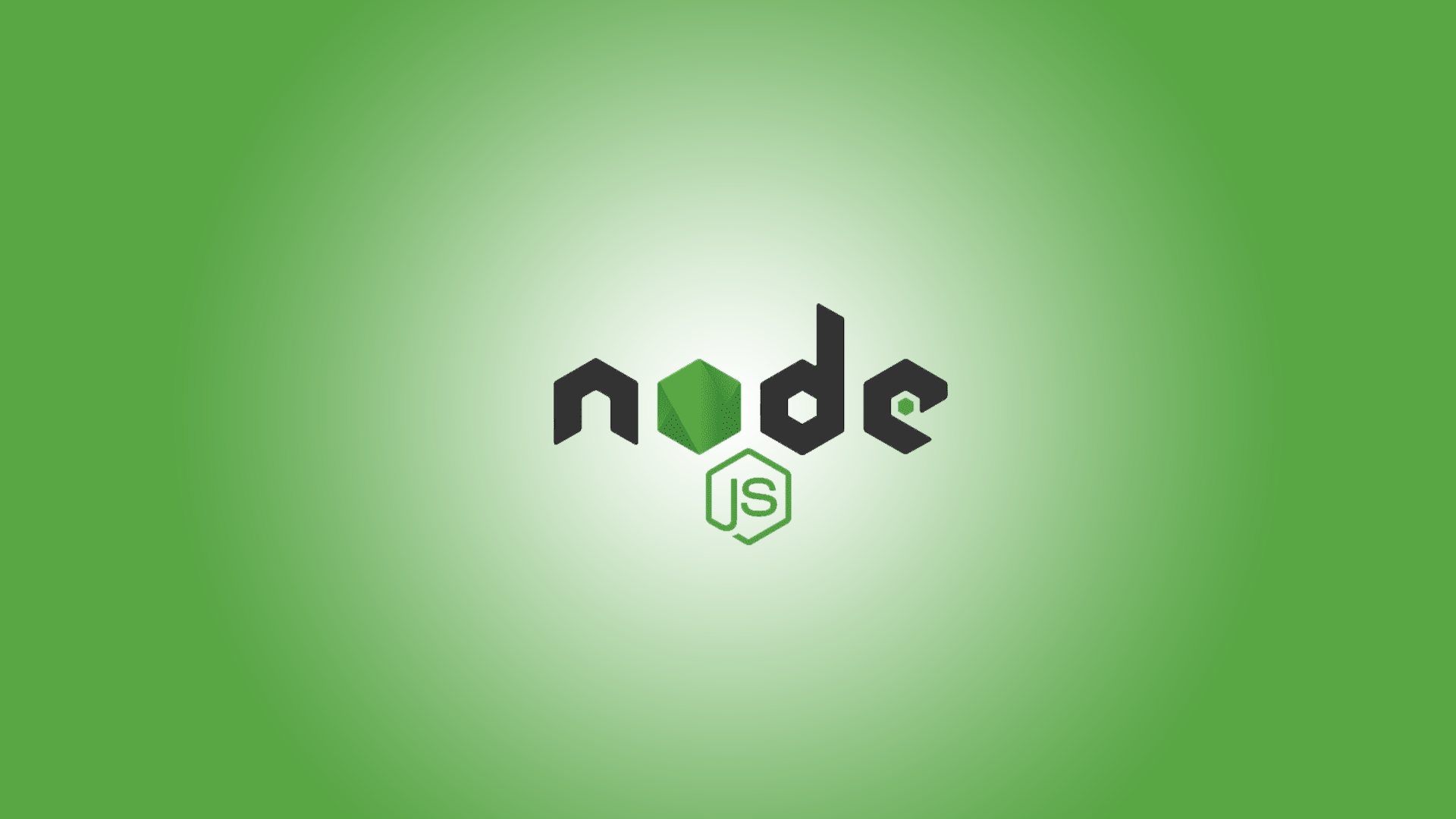
Setting Up a Node.js Project using Typescript
Creating a Node.js application using TypeScript involves a few steps to set up the project, configure TypeScript, and write TypeScript code that will eventually compile to JavaScript. Here's a step-by-step guide:
Step 1: Setting Up a Node.js Project
Initialize a new Node.js project:
mkdir ts-node-app
cd ts-node-app
npm init -y
Install necessary dependencies:
npm install typescript ts-node @types/node
Step 2: TypeScript Configuration
Create a tsconfig.json file:
npx tsc --init
This command generates a tsconfig.json file with default settings.
Configure tsconfig.json for Node.js:
Modify tsconfig.json to have appropriate settings. For example:
{
"name": "ts-node-app",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"dev": "npx ts-node ./src/app.ts",
"build": "npx tsc",
"start": "node ./dist/src/app.js"
},
"keywords": [],
"author": "",
"license": "ISC",
"devDependencies": {
"@types/node": "^20.10.3",
"nodemon": "^3.0.2",
"typescript": "^5.3.2"
}
}
- "target": "es6": Specifies the ECMAScript version to transpile to.
- "module": "commonjs": Generates Node.js-compatible module.exports.
- "strict": true: Enables strict type checking.
- "esModuleInterop": true: Simplifies interoperability between CommonJS and ES6 modules.
Step 3: Folder Structure and Code
Create a src directory:
mkdir src
Write TypeScript code:
Example src/index.ts:
const greet = (name: string): string => {
return `Hello, ${name}!`;
};
console.log(greet("World"));
Step 4: Building and Running the TypeScript Application
Compile TypeScript to JavaScript:
npx tsc
This command compiles .ts files in the src directory to .js files.
Run the application:
node ./dist/index.js
Ensure you point to the compiled JavaScript file (index.js in this example) in the dist (or appropriate) directory.
Step 5: Running TypeScript Files Directly
Alternatively, you can use ts-node to directly execute TypeScript files during development without explicitly compiling them:
npx ts-node ./src/index.ts
Additional Steps
- Development with Watch Mode: Run TypeScript in watch mode to automatically recompile files on changes:
- Using External Libraries: Install TypeScript type definitions for external packages:
npm install @types/package-name
This basic setup can be extended based on the complexity and requirements of your Node.js application. It allows you to start building a Node.js app using TypeScript, benefiting from strong typing and enhanced code maintenance.