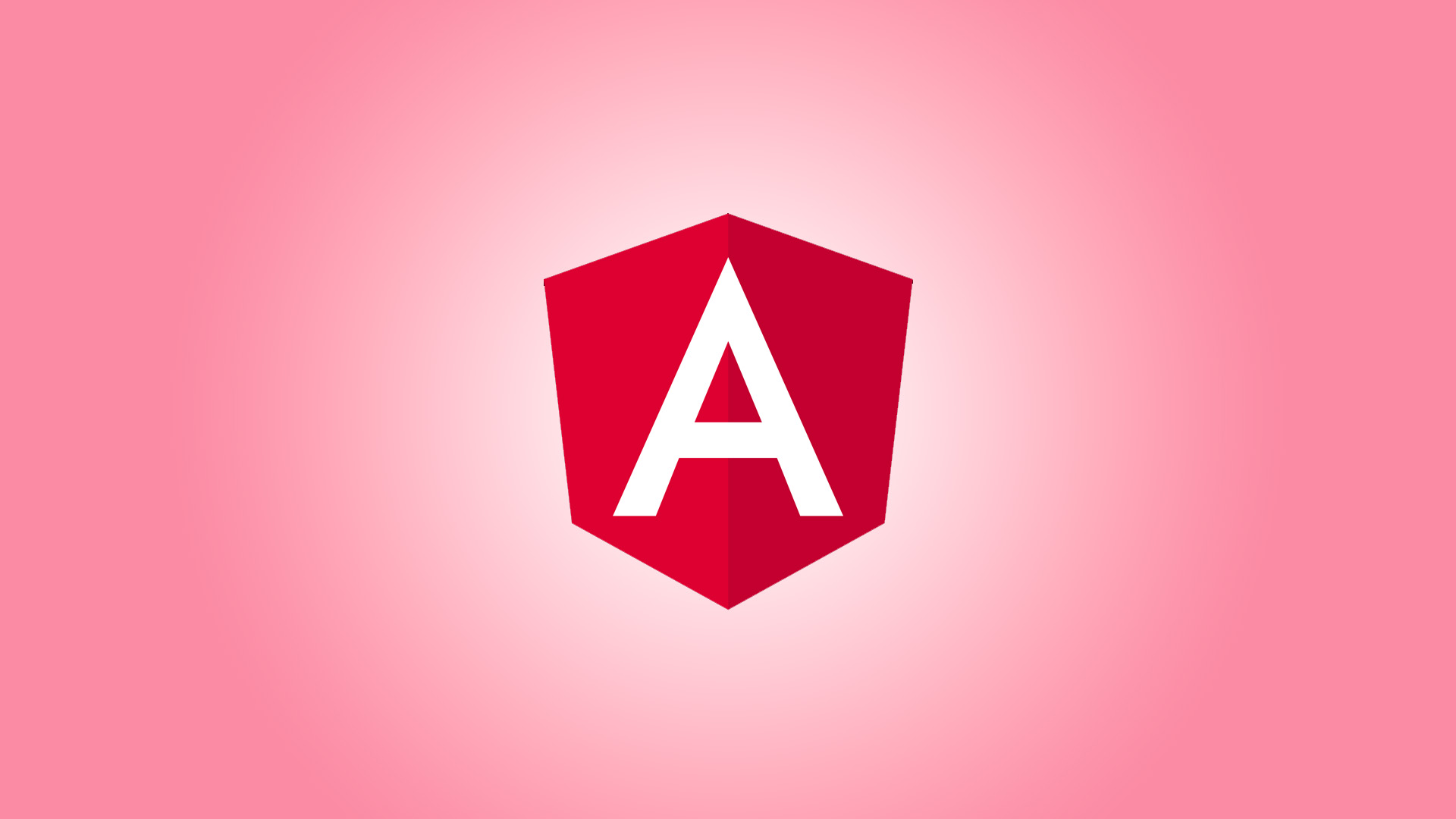
Quick Tips for Debugging Angular Applications
Here are some quick tips for debugging Angular applications along with examples:
1. Use Angular DevTools
Angular DevTools is an official browser extension that helps you inspect Angular applications. It allows you to view the component tree, check component states, and profile performance.
Example:
- Install Angular DevTools from the Chrome Web Store.
- Open your application in Chrome, and use the Angular DevTools panel to inspect your component tree and data bindings.
2. Check Console Logs
Console logs can provide valuable insights into what’s happening in your application. Use console.log()
statements strategically to track down issues.
Example:
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
})
export class ExampleComponent {
data: any;
ngOnInit() {
this.loadData();
}
loadData() {
console.log('Loading data...');
this.data = [/* some data */];
console.log('Data loaded:', this.data);
}
}
3. Utilize Source Maps
Source maps help you debug TypeScript code by mapping it to the original source. Make sure source maps are enabled in your Angular application.
Example:
- Ensure
sourceMap: true
is set in yourangular.json
file under the build options:"build": { "options": { "sourceMap": true } }
- This allows you to debug TypeScript directly in your browser’s developer tools.
4. Leverage Angular’s Built-in Debugging Tools
Angular provides built-in debugging tools like ng.probe()
to inspect Angular internals. However, use these with caution as they can expose sensitive application details.
Example:
import { Component, OnInit } from '@angular/core';
import { NgProbeToken } from '@angular/core';
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
})
export class ExampleComponent implements OnInit {
data: any;
ngOnInit() {
const probe = ng.probe(document.querySelector('app-example'));
console.log(probe.componentInstance);
}
}
5. Use Breakpoints in Developer Tools
Set breakpoints in your TypeScript files using browser developer tools. This allows you to pause execution and inspect the state of your application at specific points.
Example:
- Open Chrome DevTools (F12 or right-click > Inspect).
- Go to the "Sources" tab and find your TypeScript files.
- Click on the line number where you want to set a breakpoint.
- Reload your application and execution will pause at the breakpoint, allowing you to inspect variables and call stack.
6. Check for Common Errors
Look out for common Angular errors such as ExpressionChangedAfterItHasBeenCheckedError
, which usually occurs when data changes after Angular's change detection has run.
Example:
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
})
export class ExampleComponent implements OnInit {
data: any;
constructor(private cd: ChangeDetectorRef) {}
ngOnInit() {
setTimeout(() => {
this.data = [/* some data */];
this.cd.detectChanges(); // Trigger change detection manually
}, 1000);
}
}
7. Inspect Network Requests
Use the Network tab in Chrome DevTools to inspect HTTP requests and responses. This helps in debugging issues related to API calls.
Example:
- Open Chrome DevTools (F12 or right-click > Inspect).
- Go to the "Network" tab.
- Reload your application and observe the network requests. Check for failed requests or unexpected responses.
8. Utilize Angular CLI Commands
Angular CLI provides commands for linting and building your application, which can help identify issues early.
Example:
- Run
ng lint
to check for linting issues. - Run
ng build --prod
to build your application for production and catch any build-time issues.
By using these tips and examples, you should be able to debug and troubleshoot Angular applications more effectively.