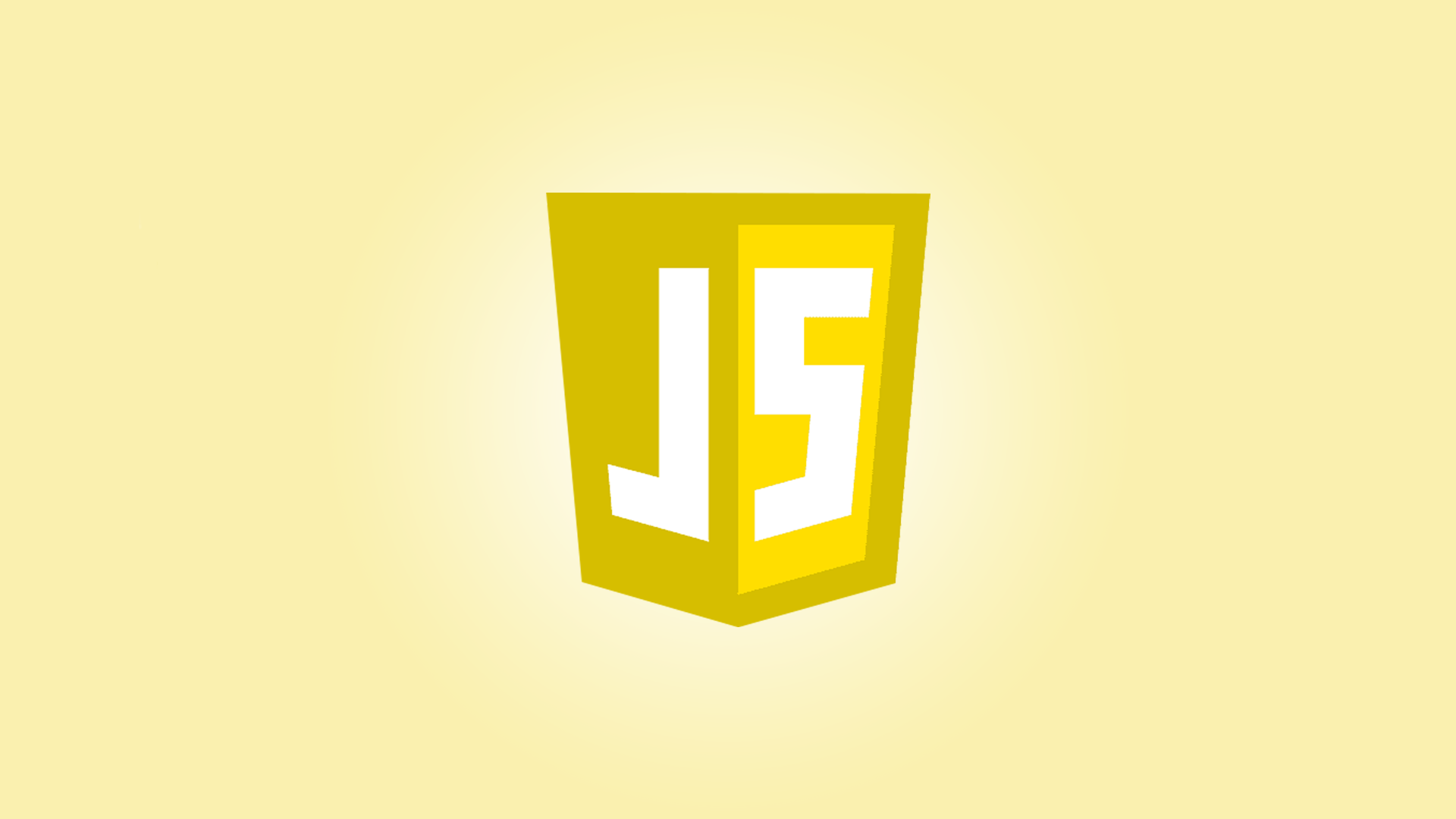
Difference between JavaScript map and forEach
Javascript
07, Nov 2023
map and forEach are both methods used to iterate over elements in a collection, such as an array, in various programming languages. However, they serve different purposes and have distinct behaviors:
-
map:
- Purpose: map is used to transform elements in a collection and create a new array with the same number of elements, but with each element modified according to a provided function.
- Return Value: map returns a new array containing the results of applying the provided function to each element in the original array.
const numbers = [1, 2, 3, 4, 5];
const squared = numbers.map((num) => num * num);
// squared is [1, 4, 9, 16, 25]
-
forEach
- Purpose: forEach is used to iterate over each element in a collection and execute a provided function for each element. It doesn't produce a new array or modify the existing one. It is often used when you want to perform some action on each element but don't need to create a new array.
- Return Value: forEach doesn't return anything (it has no return value), and it doesn't modify the original array.
const numbers = [1, 2, 3, 4, 5];
numbers.forEach((num) => {
console.log(num);
});
// This will log each number in the array.
In summary, the main difference between map and forEach is in their purpose and return value. Use map when you want to transform elements and create a new array, and use forEach when you want to iterate over elements and perform some action on each element without creating a new array.