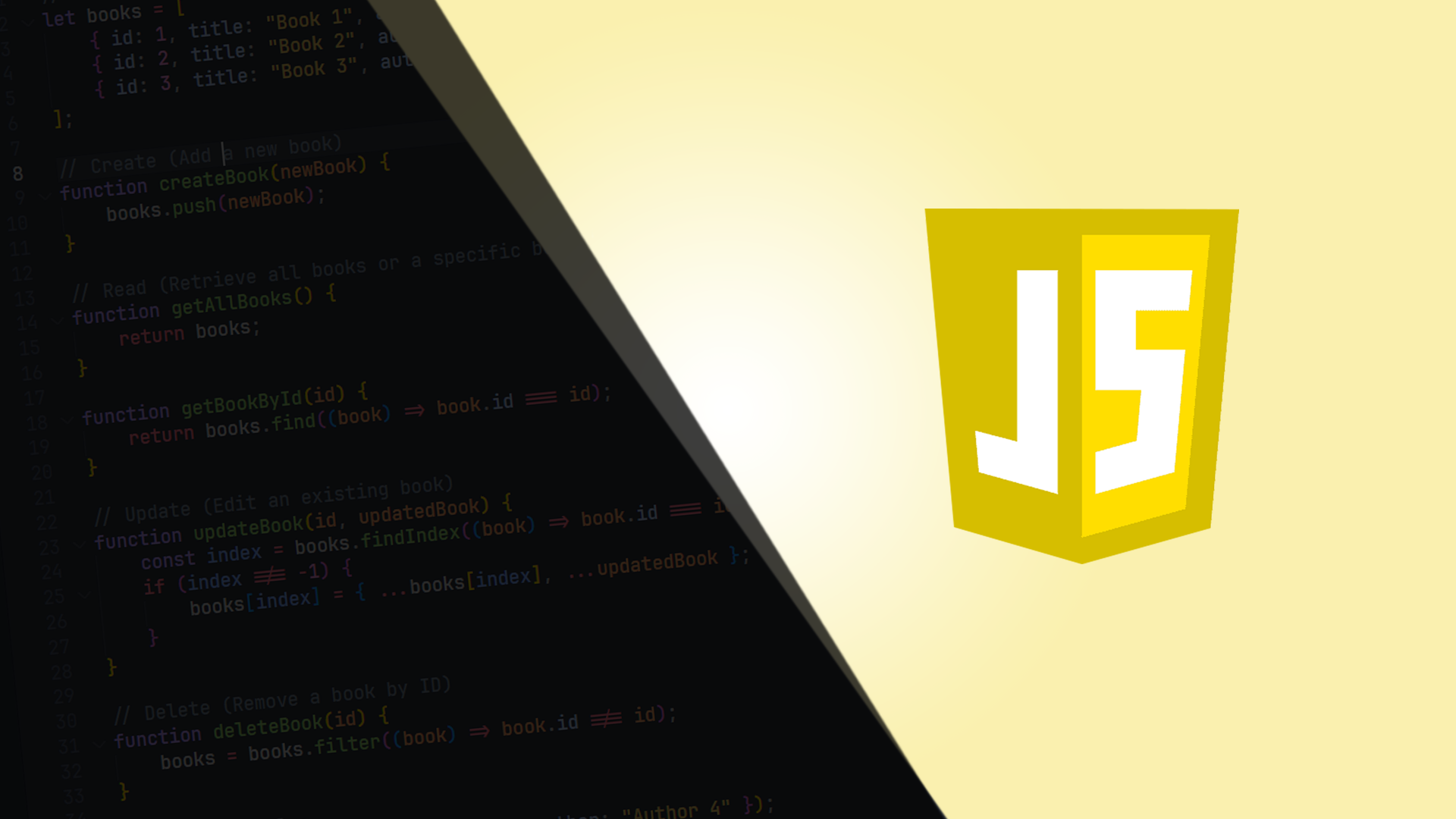
Difference between JavaScript map and filter
Javascript
07, Nov 2023
map and filter are two higher-order functions commonly used in programming, particularly in functional programming languages like Python, JavaScript, and many others. They serve different purposes and have distinct behaviors:
-
map:
- Purpose: map is used to transform a collection (e.g., a list or array) by applying a function to each element of the collection and returning a new collection containing the results of those function applications.
- Return Value: returns a new collection of the same length as the input collection, where each element is the result of applying the given function to the corresponding element of the input collection.
const numbers = [1, 2, 3, 4, 5]
const squared_numbers = list(map(lambda x: x**2, numbers))
// squared_numbers will be [1, 4, 9, 16, 25]
-
filter
- Purpose: filter is used to select elements from a collection that satisfy a certain condition (as specified by a given function) and return a new collection containing only those elements.
- Return Value: filter returns a new collection that includes only the elements from the input collection for which the given function returns True.
const numbers = [1, 2, 3, 4, 5]
const even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
// even_numbers will be [2, 4]
In summary, map is used for transforming elements of a collection, while filter is used for selecting elements from a collection based on a condition. Both functions are valuable tools for working with collections, and they can often be used in combination to perform more complex data manipulations.