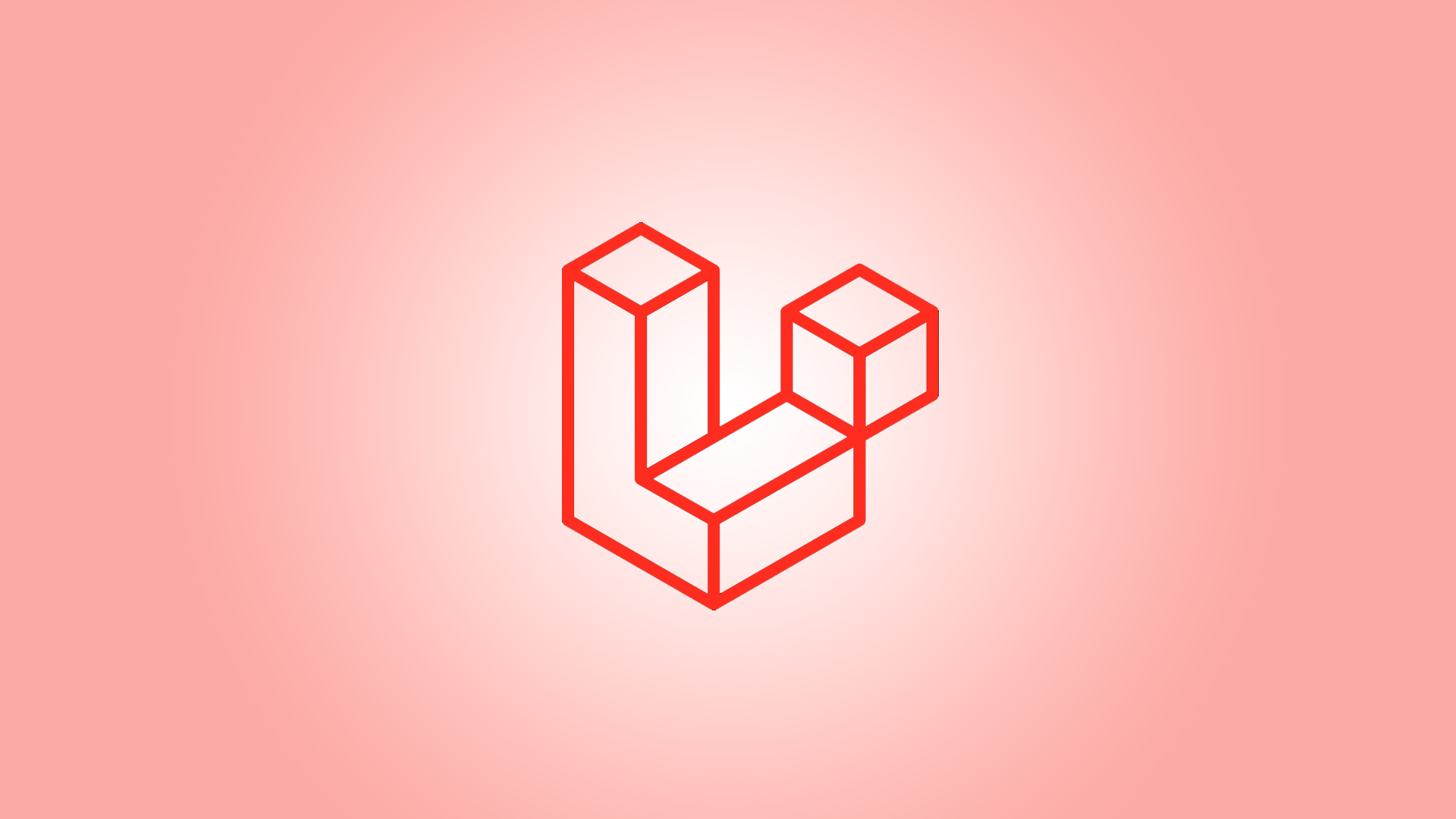
Create Laravel 10 and Vue 3 project with Vite
Laravel with Vue.js is a powerful combination for building modern web applications. Here's a short note on how these two technologies work together:
Laravel: Laravel is a robust PHP web application framework known for its back-end capabilities. It provides features for routing, authentication, database management, and more. Laravel can serve as the foundation for your web application's server-side logic and API endpoints.
Vue.js: Vue.js is a progressive JavaScript framework for building user interfaces. It excels in creating interactive, dynamic, and responsive front-end components. Vue.js allows you to build the user interface of your application, handling tasks like data binding, event handling, and component-based architecture.
First of all, create new Laravel project using below command:
composer create-project laravel/laravel laravel-vue-app
laravel new laravel-vue-app
cd laravel-vue-app
Now, Install npm packages using below command
npm install
npm install vue@next vue-loader@next
npm i @vitejs/plugin-vue
Now, edit file vite.config.js
import { defineConfig } from 'vite';
import laravel from 'laravel-vite-plugin';
import vue from '@vitejs/plugin-vue'
export default defineConfig({
plugins: [
vue(),
laravel({
input: ['resources/css/app.css', 'resources/js/app.js'],
refresh: true,
}),
],
});
Now, place below code inside resources/js/app.js file
import {createApp} from 'vue'
import App from '../vue/App.vue'
createApp(App).mount("#app")
Now, replace below code in resources/views/welcome.blade.php
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>ًApplication</title>
@vite('resources/css/app.css')
</head>
<body>
<div id="app"></div>
@vite('resources/js/app.js')
</body>
</html>
Create, App.vue file inside resources/vue folder with below code
<template>
<div>Vue Application</div>
</template>
Now, run project using below commands:
php artisan serve
npm run dev